给你二叉树的根节点 root 和一个整数目标和 targetSum ,找出所有 从根节点到叶子节点 路径总和等于给定目标和的路径。
叶子节点 是指没有子节点的节点。
示例 1:
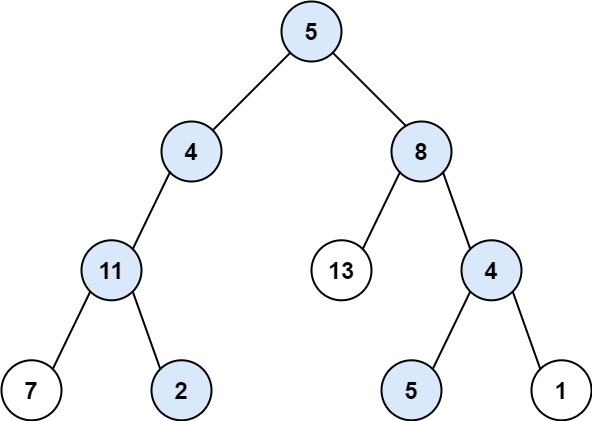
输入:root = [5,4,8,11,null,13,4,7,2,null,null,5,1], targetSum = 22
输出:[[5,4,11,2],[5,8,4,5]]
思路:回溯思想+DFS实现,因为要返回所有的路径,因此用path记录当前遍历的路径。当遍历到叶节点并且节点的值等于targetSum时,即找到符合条件的路径。
遍历时更新targetSum为targetSum - 当前节点的value
注意回溯时将路径path中最后一个节点移除。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
|
class Solution { public List<List<Integer>> pathSum(TreeNode root, int targetSum) { List<List<Integer>> ans = new ArrayList<>(); if (root == null) { return ans; } Deque<Integer> path = new LinkedList<>(); dfs(root, ans, path, targetSum); return ans; }
private void dfs(TreeNode root, List<List<Integer>> ans, Deque<Integer> path, int targetSum) { if (root == null) { return; } if (root.left == null && root.right == null && root.val == targetSum) { path.addLast(root.val); ans.add(new ArrayList(path)); path.removeLast(); return; } path.addLast(root.val); dfs(root.left, ans, path, targetSum - root.val); dfs(root.right, ans, path, targetSum - root.val); path.removeLast(); } }
|